In this post, I will show you how to implement Spring JPA One-to-Many unidirectional mapping with Hibernate in Spring Boot with an example using @OneToMany annotation.
What you need
- JDK 8+ or Open JDK 8+
- Spring Boot 2.7.2 or above
- Maven 3+
- MySql Server 5+
- Your favorite IDE
What you will learn
- How to set up a Spring Boot project.
- How to define Data Models and Repository interfaces.
- How to configure Spring Data, JPA, and Hibernate to work with different databases.
- How to use the CommandLineRunner interface to add data on application start-up.
- How to use @OneToMany and @ManyToOne annotation.
Let’s consider an application where the idea is to map the model of a college with students taking courses given by teachers. Here is what this model looks like:
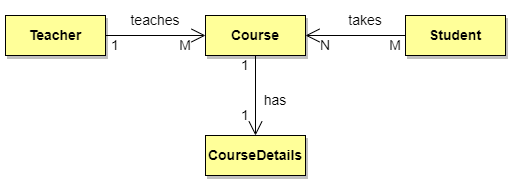
As we can see, there are a few classes. These classes have relationships between them. In this post, we will be taking only the Teacher and Course classes. By the end of this post, we’ll have mapped those classes to database tables, preserving their relationships.
We’re gonna create a Spring Boot project from scratch, then we implement JPA/Hibernate One to Many Unidirectional Mapping with the teachers and the courses table as follows:
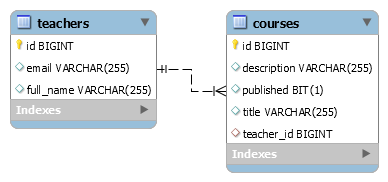
Also, check JPA / Hibernate One to One Mapping Example with Spring Boot
Project Structure
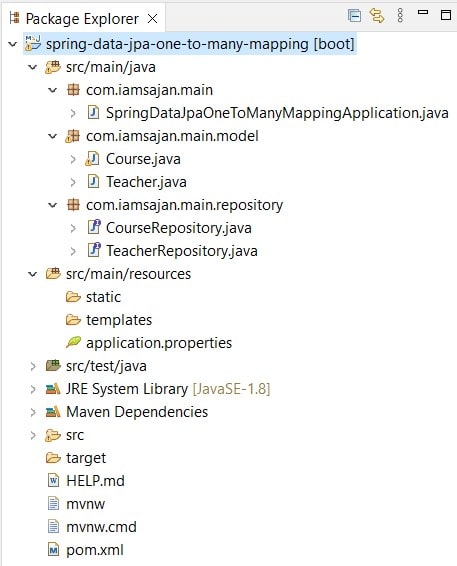
Project Dependencies
We will use the following dependencies.
- spring-boot-starter-data-jpa
- spring-boot-starter-web
- mysql-connector-java
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
Configure Spring Datasource, JPA, Hibernate
Under the src/main/resources folder, open application.properties to configure the Spring Datasource JDBC URL, username, and password of your local MySql Server.
#Database configuration sql
spring.datasource.url= jdbc:mysql://localhost:3306/testdb?useSSL=false
spring.datasource.username= root
spring.datasource.password=
#Hibernate configuration
spring.jpa.hibernate.ddl-auto= update
spring.jpa.properties.hibernate.dialect= org.hibernate.dialect.MySQL8Dialect
Create the testdb database in your local MySQL server if not exists. That is all that we need to do with the database server.
We don’t have to create table schemas, the spring.jpa.hibernate.ddl-auto=update configuration allows JPA and Hibernate does that based on the entity-relationship mappings.
spring.jpa.properties.hibernate.dialect is optional and can be used to specify the current database dialect to let it generate better SQL queries for that database.
Defining the Models
Let’s now define the model(entities) classes mapped to the tables we saw earlier.
- Teacher Model(Entity)
The Teacher model class has four fields: id, fullName, email, and courses. Create the model package and then the Teacher class inside the model package with the following contents.
package com.iamsajan.main.model;
import java.util.List;
import javax.persistence.*;
@Entity
@Table(name = "teachers")
public class Teacher {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(name = "full_name")
private String fullName;
@Column(name = "email")
private String email;
@OneToMany
@JoinColumn(name = "teacher_id", referencedColumnName = "id")
private List<Course> courses;
public Teacher() {
}
public Teacher(String fullName, String email, List<Course> courses) {
this.fullName = fullName;
this.email = email;
this.courses = courses;
}
// Getters and Setters (Omitted for brevity)
// You can generate getter and setter using shortcut key Alt+Shift+s on Eclipse
// and STS
}
- Course Model(Entity)
The Course model class has four fields: id, title, description and published. Create the Course class inside the model package with the following contents.
package com.iamsajan.main.model;
import java.io.Serializable;
import javax.persistence.*;
@Entity
@Table(name = "courses")
public class Course implements Serializable{
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
@Column(name = "title")
private String title;
@Column(name = "description")
private String description;
@Column(name = "published")
private boolean published;
// Hibernate requires a no-argument constructor
public Course() {
}
public Course(String title, String description, boolean published) {
this.title = title;
this.description = description;
this.published = published;
}
// Getters and Setters (Omitted for brevity)
// You can generate getter and setter using shortcut key Alt+Shift+s on Eclipse
// and STS
}
Defining the Repositories
Let’s now define the repositories interfaces for Teacher and Course as CourseRepository and TeacherRepository. Both interfaces extend the repositories from Spring Data JPA’s JpaRepository interface.
- TeacherRepository
Create the repository package and then the TeacherRepository interface inside the repository package with the following contents.
package com.iamsajan.main.repository;
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.stereotype.Repository;
import com.iamsajan.main.model.Teacher;
@Repository
public interface TeacherRepository extends JpaRepository<Teacher, Long> {
}
- CourseRepository
Create the CourseRepository interface inside the repository package with the following contents.
package com.iamsajan.main.repository;
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.stereotype.Repository;
import com.iamsajan.main.model.Course;
@Repository
public interface CourseRepository extends JpaRepository<Course, Long> {
}
That’s all we need to do because of SimpleJpaRepository, Which implements all the methods to perform CRUD operations on Teacher and Course entities. JpaRepository interface called SimpleJpaRepository which is plugged in at runtime.
Creating data with JPA and Hibernate
I’ve implemented CommandLineRunner interface in the main class to run some code once the application has started. Open your main class. In my case (SpringDataJpaOneToManyMappingApplication.java) and add the following contents.
package com.iamsajan.main;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.CommandLineRunner;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import com.iamsajan.main.model.Course;
import com.iamsajan.main.model.Teacher;
import com.iamsajan.main.repository.CourseRepository;
import com.iamsajan.main.repository.TeacherRepository;
@SpringBootApplication
public class SpringDataJpaOneToManyMappingApplication implements CommandLineRunner {
@Autowired
private TeacherRepository teacherRepository;
@Autowired
private CourseRepository courseRepository;
public static void main(String[] args) {
SpringApplication.run(SpringDataJpaOneToManyMappingApplication.class, args);
}
@Override
public void run(String... args) throws Exception {
// Create Course instances
Course course1 = new Course("Spring Boot", "Spring Boot Jpa Hibernate one to many mapping", true);
Course course2 = new Course("Angular", "Advance Angular Programming", true);
Course course3 = new Course("React", "Advance React Programming", true);
// Save Courses
courseRepository.save(course1);
courseRepository.save(course2);
courseRepository.save(course3);
// Create Teacher instance
Teacher teacher = new Teacher("Sajan K.C.", "[email protected]", List.of(course1, course2, course3));
// Save Teacher with Courses
teacherRepository.save(teacher);
}
}
You can also clean up your database tables by adding the following line of code inside the run method. Also, don’t forget to Autowire CourseRepository.
// Clean up database tables
teacherRepository.deleteAllInBatch();
courseRepository.deleteAllInBatch();
Run and Test
Right-click on the project and select Run as > Java Application.
Or you can use a terminal to run the application from the root directory as follows:
mvn spring-boot:run
Conclusion
Today we’ve built a Spring Boot example using Spring Data JPA, and Hibernate One to Many relationships with MySQL.
Source code
In my GitHub repository, you can find the source code for the sample project we build in this post.
Keywords: onetomany example, spring boot jpa one to many example, spring boot one to many, one to many jpa example, jpa onetomany example, hibernate one to many mapping, onetomany jpa example, onetomany spring boot, jpa one to many example, many to one spring boot, jpa many to one example, spring jpa one to many example, jpa one to many mapping example, spring boot many to many, many to one hibernate, spring jpa many to one, jpa many to one, spring boot jpa one to many example, spring jpa one to many example, spring boot one to many, spring jpa one to many, one to many jpa example, onetomany spring boot, pa many to one example, jpa one to many example.
Pingback: Spring boot + Spring Data JPA + PostgreSQL example - Sajan K.C.